ADC Example : 0-5V Volt Meter
C language example code Volt meter for Analog to Digital converter in Bootloader USB PIC18F4550 kit.
Compiler : Microchip C18 C Compiler
Kit : Bootloader USB PIC18F4550
Project : 0-5V Voltmeter
LCD Details
Hardware connection : connect POT1 and RA0
Voltmeter output
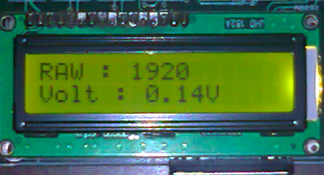
Source Code
#include < p18F4550.h>
#include < stdlib.h>
#include < delays.h>
#include "bootlcd.h"
void low_isr(void);
void high_isr(void);
#pragma code low_vector=0x2018
void interrupt_at_low_vector(void)
{
_asm GOTO low_isr _endasm
}
#pragma code
#pragma code high_vector=0x2008
void interrupt_at_high_vector(void)
{
_asm GOTO high_isr _endasm
}
#pragma code
#pragma interruptlow low_isr
void low_isr (void)
{
return;
}
#pragma interrupt high_isr
void high_isr (void)
{
return;
}
void DelayFor18TCY( void )
{
Delay10TCYx(2); // 5us delay
return;
}
void DelayPORXLCD (void)
{
Delay1KTCYx(75); // Delay of 15ms
// Cycles = (TimeDelay * Fosc) / 4
// Cycles = (15ms * 20MHz) / 4
// Cycles = 75,000
return;
}
void DelayXLCD (void)
{
Delay1KTCYx(25); // Delay of 5ms
// Cycles = (TimeDelay * Fosc) / 4
// Cycles = (5ms * 20MHz) / 4
// Cycles = 25,000
return;
}
void display_lcd (unsigned int intval)
{
unsigned int volt;
char out[10];
volt = intval / 131 ;
while(BusyXLCD()); // Wait if LCD busy
SetDDRamAddr(0x00);
putrsXLCD("RAW : ");
ltoa(intval, out);
putsXLCD(out);
putrsXLCD(" ");
while(BusyXLCD()); // Wait if LCD busy
SetDDRamAddr(0x40); // select 2 line
putrsXLCD("Volt : ");
itoa(volt, out);
while(BusyXLCD()); // Wait if LCD busy
if(volt>99)
{
WriteDataXLCD(out[0]);
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD('.');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD(out[1]);
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD(out[2]);
}else if(volt>9)
{
WriteDataXLCD('0');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD('.');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD(out[0]);
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD(out[1]);
}
else
{
WriteDataXLCD('0');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD('.');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD('0');
while(BusyXLCD()); // Wait if LCD busy
WriteDataXLCD(out[0]);
}
putrsXLCD("V ");
}
void main( void )
{
char temdata;
unsigned int current_ad_value;
TRISAbits.TRISA0 = 1;
/* FOSC/32 clock select , left alignment*/
ADCON2bits.ADCS0 = 1;
ADCON2bits.ADCS1 = 1;
ADCON2bits.ADCS2 = 1;
ADCON2bits.ADFM = 0;
/* interal ref */
ADCON1 = 0b00000000;
/* Turn on the ADC */
ADCON0bits.ADON = 1;
OpenXLCD(); // configure external LCD
while(1)
{
/* Give the ADC time to get ready. */
Delay100TCYx (2);
/* start the ADC conversion */
ADCON0bits.GO = 1;
while (ADCON0bits.GO);
current_ad_value = ADRES;
display_lcd(current_ad_value);
Delay10KTCYx (200);
}
}
User Comments
No Posts found !Login to Post a Comment.