LCD Display circuit
An HD44780 Character LCD is a de facto industry standard liquid crystal display (LCD) display device designed for interfacing with embedded systems. These screens come in a variety of configurations including 8x1, which is one row of eight characters, 16x2, and 20x4. The most commonly manufactured configuration is 40x2 characters
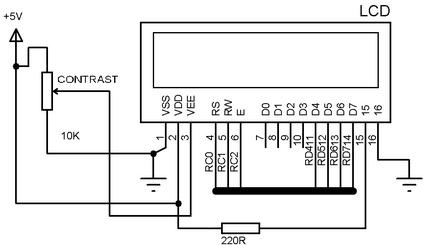
Character LCDs use a standard 14-pin interface and those with backlights have 16 pins. The pinouts are as follows:
Ground
VCC (+3.3 to +5V)
Contrast adjustment (VO)
Register Select (RS). RS=0: Command, RS=1: Data
Read/Write (R/W). R/W=0: Write, R/W=1: Read
Clock (Enable). Falling edge triggered
Bit 0 (Not used in 4-bit operation)
Bit 1 (Not used in 4-bit operation)
Bit 2 (Not used in 4-bit operation)
Bit 3 (Not used in 4-bit operation)
Bit 4
Bit 5
Bit 6
Bit 7
Backlight Anode (+)
Backlight Cathode (-)
There may also be a single backlight pin, with the other connection via Ground or VCC pin. The two backlight pins may precede the pin 1.
The nominal backlight voltage is around 4.2V at 25ËšC using a VDD 5V capable model. Character LCDs can operate in 4-bit or 8-bit mode. In 4 bit mode, pins 7 through 10 are unused and the entire byte is sent to the screen using pins 11 through 14 by sending 4-bits (nibble) at a time.
Function Details in bootlcd.h file
Function Name: OpenXLCD
Return Value: void
Description: This routine configures the LCD. Based on the Hitachi HD44780 LCD controller. The routine will configure the I/O pins of the microcontroller, setup the LCD for 4- or 8-bit mode and clear the display. The user must provide three delay routines: DelayFor18TCY() provides a 18 Tcy delay. DelayPORXLCD() provides at least 15ms delay. DelayXLCD() provides at least 5ms delay.
void OpenXLCD()
{
// The data bits must be either a 8-bit port or the upper or
// lower 4-bits of a port. These pins are made into inputs
// Upper 4-bits of the port
DATA_PORT &= 0x0f;
TRIS_DATA_PORT &= 0x0F;
TRIS_RW = 0; // All control signals made outputs
TRIS_RS = 0;
TRIS_E = 0;
RW_PIN = 0; // R/W pin made low
RS_PIN = 0; // Register select pin made low
E_PIN = 0; // Clock pin made low
// Delay for 15ms to allow for LCD Power on reset
Delay1KTCYx(75); // Delay of 15ms
//-------------------reset procedure through software----------------------
WriteCmdXLCD(0x30);
Delay10KTCYx(0x05);
WriteCmdXLCD(0x30);
Delay10KTCYx(0x01);
WriteCmdXLCD(0x32);
while( BusyXLCD() );
//------------------------------------------------------------------------------------------
// Set data interface width, # lines, font
while(BusyXLCD()); // Wait if LCD busy
WriteCmd8bit(FOUR_BIT & LINES_5X7); // Function set cmd
while(BusyXLCD());
//WriteCmdXLCD(LINES_5X7);
// Turn the display on then off
Delay10KTCYx(0x05); // Wait if LCD busy
WriteCmdXLCD(DOFF&CURSOR_OFF&BLINK_OFF); // Display OFF/Blink OFF
while(BusyXLCD()); // Wait if LCD busy
WriteCmdXLCD(DON&CURSOR_OFF&BLINK_OFF); // Display ON/Blink ON
// Clear display
while(BusyXLCD()); // Wait if LCD busy
WriteCmdXLCD(0x01); // Clear display
// Set entry mode inc, no shift
while(BusyXLCD()); // Wait if LCD busy
WriteCmdXLCD(SHIFT_DISP_LEFT); // Entry Mode
// Set DD Ram address to 0
while(BusyXLCD()); // Wait if LCD busy
SetDDRamAddr(0x80); // Set Display data ram address to 0
return;
}
Function Name: SetCGRamAddr
Return Value: void
Parameters: CGaddr: character generator ram address
Description: This routine sets the character generator address of the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine.
void SetCGRamAddr(unsigned char CGaddr)
{
// Upper nibble interface
TRIS_DATA_PORT &= 0x0f; // Make nibble input
DATA_PORT &= 0x0f; // and write upper nibble
DATA_PORT |= ((CGaddr | 0b01000000) & 0xf0);
RW_PIN = 0; // Set control signals
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock cmd and address in
DelayFor18TCY();
E_PIN = 0;
DATA_PORT &= 0x0f; // Write lower nibble
DATA_PORT |= ((CGaddr<<4)&0xf0);
DelayFor18TCY();
E_PIN = 1; // Clock cmd and address in
DelayFor18TCY();
E_PIN = 0;
TRIS_DATA_PORT |= 0xf0; // Make inputs
return;
}
Function Name: SetDDRamAddr
Return Value: void
Parameters: CGaddr: display data address
Description: This routine sets the display data address
of the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine.
void SetDDRamAddr(unsigned char DDaddr)
{
// 4-bit interface
// Upper nibble interface
TRIS_DATA_PORT &= 0x0f; // Make port output
DATA_PORT &= 0x0f; // and write upper nibble
DATA_PORT |= ((DDaddr | 0b10000000) & 0xf0);
RW_PIN = 0; // Set control bits
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock the cmd and address in
DelayFor18TCY();
E_PIN = 0;
// Upper nibble interface
DATA_PORT &= 0x0f; // Write lower nibble
DATA_PORT |= ((DDaddr<<4)&0xf0);
DelayFor18TCY();
E_PIN = 1; // Clock the cmd and address in
DelayFor18TCY();
E_PIN = 0;
// Upper nibble interface
TRIS_DATA_PORT |= 0xf0; // Make port input
return;
}
Function Name: BusyXLCD
Return Value: char: busy status of LCD controller
Parameters: void
Description: This routine reads the busy status of the Hitachi HD44780 LCD controller.
unsigned char BusyXLCD(void)
{
RW_PIN = 1; // Set the control bits for read
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock in the command
DelayFor18TCY();
// Upper nibble interface
if(DATA_PORT&0x80)
{
E_PIN = 0; // Reset clock line
DelayFor18TCY();
E_PIN = 1; // Clock out other nibble
DelayFor18TCY();
E_PIN = 0;
RW_PIN = 0; // Reset control line
return 1; // Return TRUE
}
else // Busy bit is low
{
E_PIN = 0; // Reset clock line
DelayFor18TCY();
E_PIN = 1; // Clock out other nibble
DelayFor18TCY();
E_PIN = 0;
RW_PIN = 0; // Reset control line
return 0; // Return FALSE
}
}
Function Name: ReadAddrXLCD
Return Value: char: address from LCD controller
Parameters: void
Description: This routine reads an address byte from the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine. The address is read from the character generator RAM or the display data RAM depending on what the previous SetxxRamAddr routine was called.
unsigned char ReadAddrXLCD(void)
{
char data; // Holds the data retrieved from the LCD
// 4-bit interface
RW_PIN = 1; // Set control bits for the read
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock data out of the LCD controller
DelayFor18TCY();
// Upper nibble interface
data = DATA_PORT&0xf0; // Read the nibble into the upper nibble of data
E_PIN = 0; // Reset the clock
DelayFor18TCY();
E_PIN = 1; // Clock out the lower nibble
DelayFor18TCY();
// Upper nibble interface
data |= (DATA_PORT>>4)&0x0f; // Read the nibble into the lower nibble of data
E_PIN = 0;
RW_PIN = 0; // Reset the control lines
return (data&0x7f); // Return the address, Mask off the busy bit
}
Function Name: ReadDataXLCD
Return Value: char: data byte from LCD controller
Parameters: void
Description: This routine reads a data byte from the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine. The data is read from the character generator RAM or the display data RAM depending on what the previous SetxxRamAddr routine was called.
char ReadDataXLCD(void)
{
char data;
// 4-bit interface
RW_PIN = 1;
RS_PIN = 1;
DelayFor18TCY();
E_PIN = 1; // Clock the data out of the LCD
DelayFor18TCY();
// Upper nibble interface
data = DATA_PORT&0xf0; // Read the upper nibble of data
E_PIN = 0; // Reset the clock line
DelayFor18TCY();
E_PIN = 1; // Clock the next nibble out of the LCD
DelayFor18TCY();
// Upper nibble interface
data |= (DATA_PORT>>4)&0x0f; // Read the lower nibble of data
E_PIN = 0;
RS_PIN = 0; // Reset the control bits
RW_PIN = 0;
return(data); // Return the data byte
}
Function Name: WriteCmdXLCD
Return Value: void
Parameters: cmd: command to send to LCD
Description: This routine writes a command to the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine.
void WriteCmdXLCD(unsigned char cmd)
{
// 4-bit interface
// Upper nibble interface
TRIS_DATA_PORT &= 0x0f;
DATA_PORT &= 0x0f;
DATA_PORT |= cmd&0xf0;
RW_PIN = 0; // Set control signals for command
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock command in
DelayFor18TCY();
E_PIN = 0;
// Upper nibble interface
DATA_PORT &= 0x0f;
DATA_PORT |= (cmd<<4)&0xf0;
DelayFor18TCY();
E_PIN = 1; // Clock command in
DelayFor18TCY();
E_PIN = 0;
// Make data nibble input
TRIS_DATA_PORT |= 0xf0;
return;
}
Function Name: WriteCmd8bit
Return Value: void
Parameters: cmd: command to send to LCD
Description: This routine writes a command to the Hitachi HD44780 LCD controller 8 bit method. The user must check to see if the LCD controller is busy before calling this routine.
void WriteCmd8bit(unsigned char cmd)
{
// 4-bit interface
// Upper nibble interface
TRIS_DATA_PORT &= 0x0f;
DATA_PORT &= 0x0f;
DATA_PORT |= cmd&0xf0;
RW_PIN = 0; // Set control signals for command
RS_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock command in
DelayFor18TCY();
E_PIN = 0;
TRIS_DATA_PORT |= 0xf0;
return;
}
Function Name: WriteDataXLCD
Return Value: void
Parameters: data: data byte to be written to LCD
Description: This routine writes a data byte to the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine. The data is written to the character generator RAM or the display data RAM depending on what the previous SetxxRamAddr routine was called.
void WriteDataXLCD(char data)
{
// Upper nibble interface
TRIS_DATA_PORT &= 0x0f;
DATA_PORT &= 0x0f;
DATA_PORT |= data&0xf0;
RS_PIN = 1; // Set control bits
RW_PIN = 0;
DelayFor18TCY();
E_PIN = 1; // Clock nibble into LCD
DelayFor18TCY();
E_PIN = 0;
// Upper nibble interface
DATA_PORT &= 0x0f;
DATA_PORT |= ((data<<4)&0xf0);
DelayFor18TCY();
E_PIN = 1; // Clock nibble into LCD
DelayFor18TCY();
E_PIN = 0;
// Upper nibble interface
TRIS_DATA_PORT |= 0xf0;
return;
}
Function Name: putsXLCD
Return Value: void
Parameters: buffer: pointer to string
Description: This routine writes a string of bytes to the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine. The data is written to the character generator RAM or the display data RAM depending on what the previous SetxxRamAddr routine was called.
void putsXLCD(char *buffer)
{
while(*buffer) // Write data to LCD up to null
{
while(BusyXLCD()); // Wait while LCD is busy
WriteDataXLCD(*buffer); // Write character to LCD
buffer++; // Increment buffer
}
return;
}
Function Name: putrsXLCD
Return Value: void
Parameters: buffer: pointer to string
Description: This routine writes a string of bytes to the Hitachi HD44780 LCD controller. The user must check to see if the LCD controller is busy before calling this routine. The data is written to the character generator RAM or the display data RAM depending on what the previous SetxxRamAddr routine was called.
void putrsXLCD(const rom char *buffer)
{
while(*buffer) // Write data to LCD up to null
{
while(BusyXLCD()); // Wait while LCD is busy
WriteDataXLCD(*buffer); // Write character to LCD
buffer++; // Increment buffer
}
return;
}
User Comments
No Posts found !Login to Post a Comment.