Seven-Segment Display
The segments in a 7-segment display are arranged to form a single digit from 0 to F as shown in the animation:
We can display a multi-digit number by connecting additional displays. Even though LCD displays are more comfortable to work with, 7-segment displays are still standard in the industry. This is due to their temperature robustness, visibility and wide viewing angle. Segments are marked with non-capital letters: a, b, c, d, e, f, g and dp, where dp is the decimal point. The 8 LEDs inside each display can be arranged with a common cathode or common anode. With a common cathode display, the common cathode must be connected to the 0V rail and the LEDs are turned on with a logic one. Common anode displays must have the common anode connected to the +5V rail. The segments are turned on with a logic zero. The size of a display is measured in millimeters, the height of the digit itself (not the housing, but the digit!). Displays are available with a digit height of 7,10, 13.5, 20, or 25 millimeters. They come in different colors, including: red, orange, and green.
The simplest way to drive a display is via a display driver. These are available for up to 4 displays. Alternatively displays can be driven by a microcontroller and if more than one display is required, the method of driving them is called "multiplexing."
The main difference between the two methods is the number of "drive lines." A special driver may need only a single "clock" line and the driver chip will access all the segments and increment the display. If a single display is to be driven from a microcontroller, 7 lines will be needed plus one for the decimal point. For each additional display, only one extra line is needed. To produce a 4, 5 or 6 digit display, all the 7-segment displays are connected in parallel. The common line (the common-cathode line) is taken out separately and this line is taken low for a short period of time to turn on the display. Each display is turned on at a rate above 100 times per second, and it will appear that all the displays are turned on at the same time. As each display is turned on, the appropriate information must be delivered to it so that it will give the correct reading. Up to 6 displays can be accessed like this without the brightness of each display being affected. Each display is turned on very hard for one-sixth the time and the POV (persistence of vision) of our eye thinks the display is turned on the whole time. Therefore, the program has to ensure the proper timing, else the unpleasant blinking of display will occur.
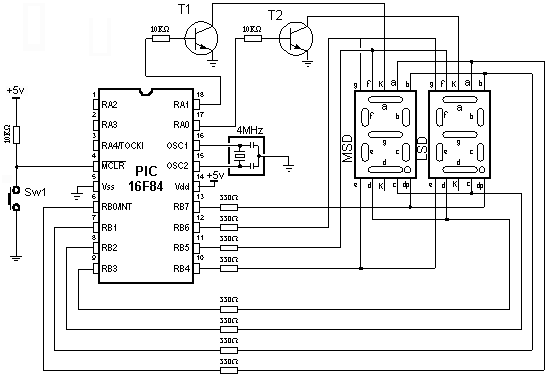
Connecting a microcontroller to 7-segment displays in multiplex mode
Program "7seg.asm" displays decimal value of a number stored in variable D.
Example:
movlw .21 | |
movlw D | ; number 21 will be printed on 7seg display |
Displaying digits is carried out in multiplex mode which means that the microcontroller alternately prints ones digit and tens digit. TMR0 interrupt serves for generating a time period, so that the program enters the interrupt routine every 5ms and performs multiplexing. In the interrupt routine, first step is deciding which segment should be turned on. In case that the tens digit was previously on, it should be turned off, set the mask for printing the ones digit on 7seg display which lasts 5ms, i.e. until the next interrupt.
For extracting the ones digit and the tens digit, macro digbyte is used. It stores the hundreds digit, the tens digit, and the ones digit into variables Dig1, Dig2, and Dig3. In our case, upon macro execution, Dig1 will equal 0, Dig2 will equal 2, and Dig3 will equal 1.
Realization of the macro is given in the following listing:
DIGBYTE MACRO PARO
LOCAL PONO
LOCAL EXITL
LOCAL EXIT2
LOCAL POZITIV
CLRF DIGL
CLRF DIG2
CLRF DIG3
POZITIV
MOVF PARO,W
MOVWF DIGTEMP
MOVLW .100
PONO
INCF DIGL,F
SUBWF DIGTEMP ,F
BTFSC STATUS, C
GOTO PONO
DECF DIGL,F
ADDWF DIGTEMP, F
EXITL
MOVLW .10
INCF DIG2,F
SUBWF DIGTEMP, F
BTFSC STATUS,C
GOTO EXITL
DECF DIG2,F
ADDWF DIGTEMP, F
EXIT2
MOVF DIGTEMP,W
MOVWF DIG3
ENDM
The following example shows the use of the macro in a program. Program prints a specified 2-digit number on a 7seg display in multiplex mode.
PROCESSOR P16F84
#INCLUDE "P16F84.INC"
__CONFIG _CP_OFF & WDT_OFF & _PWRTE_ON & _XT_OSC
ORG 0X00
GOTO MAIN ;Beginning of the program
ORG 0X04
GOTO ISR ;Beginning of the interrupt routine
Cblock 0x0c
Digl ;Variable for storing the hundreds digit of number D
Dig2 ;Variable for storing the tens digit of number D
Dig3 ;Variable for storing the ones digit of number D
Digtemp
D ;D stores the number to be displayed
One ;Auxiliary variable for multiplex disp.
W_temp ;Auxiliary variable
endc
#include "romux_lib.inc"
BANKSEL TRISA
MOVLW B'11111100' ;RA0 and RAl are output pins used for
MOVWF TRISA ;multip lexing
CLRF TRISB ;Port B is output
MOVLW B'10000100' ;Prescaler is 32 meaning that TMR 0 is
MOVWF OPTI0N_REG ;incremented every 32ms supposition that
;4HHz oscilator is used
BANKSEL PORTA
MOVLW .96 ;Starting value of THRO is 96,
MOVWF TMRO ;interrupt occurs every (255-97)*32us=5.088ms
MOVLW B'10100000' ;TMR0 interrupt enabled
MOVWF INTC0N
MOVLW .21 ;Print decimal value 21
MOVWF D
CLRF ONE
CLRF PORTA ;Turn off both displays at the start
LOOP
GOTO LOOP ;Main loop
ISR
MOVWF W_TEMP ;Store the contents of W register
MOVLW .96 ;
MOVWF TMRO ;initialize THRO to have the next interrupt in
;approximately 5ms
BCF INTC0N,T0IF ;Clear flag overflow TMRO to be able
;to react to the next interrupt
BCF PORTA,0 ;Turn off both displays
BCF PORTA,1
MOVF ONE,F
BTFSC STATUS,Z ;Which display should be on?
GOTO MSDON ;
LSDON ;If HSD was previously on,
BCF ONE,0 ;0ne stores that LSD is on
MOVLW HIGH BCDTO7SEG;Prior to jump to lookup table, PCLATH
MOVWF PCLATH ;register should be initialized with the higher
;byte of the address lookup (HIGH lookup)
;to prevent errors in addressing
DIGBYTE D ;Macro for separating digits of number D
MOVF DIG3,RA ;Dig3 holds the loner digit
CALL BCDTO7SEG ;Call the lookup table from which the
;appropriate mask is read, for the digit
MOVWF PORTB ;to be printed on 7-seg display raith common
;cathode
BSF PORTA, 1 ;Turn on LSD display (remain on for 5ms,
;until the next TMRO interrupt)
MOVF W_TEMP ,RA ;W registrer has the same value it had before
;entering the interrupt subroutine
RETFIE
MSDON
BSF ONE,0 ;0ne stores that HSD is on
MOVLW HIGH BCDTO7SEG ;Initializing PCLATH register before using
movwf PCLATH ;addwf PCL,f instruction (when returning from
;routine, 'call' does it automatically)
DIGBYTE D ;Macro for separating digits of number D
MOVF DIG2,RA ;HI holds the higher digit
CALL BCDTO7SEG ;Set the appropriate mask for number HI to
;P0RTB
MOVWF P0RTB
BSF PORTA,0 ;Turn on display for printing the more
;significant digit
MOVF W_TEMP,W
RETFIE
ORG 0X300 ;Lookup table is located at the beginning
;of the third page, other locations are
;possible as long as the entire table is on
;single page
BCDTO7SEG
ADDWF PCL,F
DT 0X3F, 0X06, 0X5B, 0X4F, 0X66, 0X6D, 0X7D, 0X07, 0X7F, 0X6F
END
User Comments
No Posts found !Login to Post a Comment.